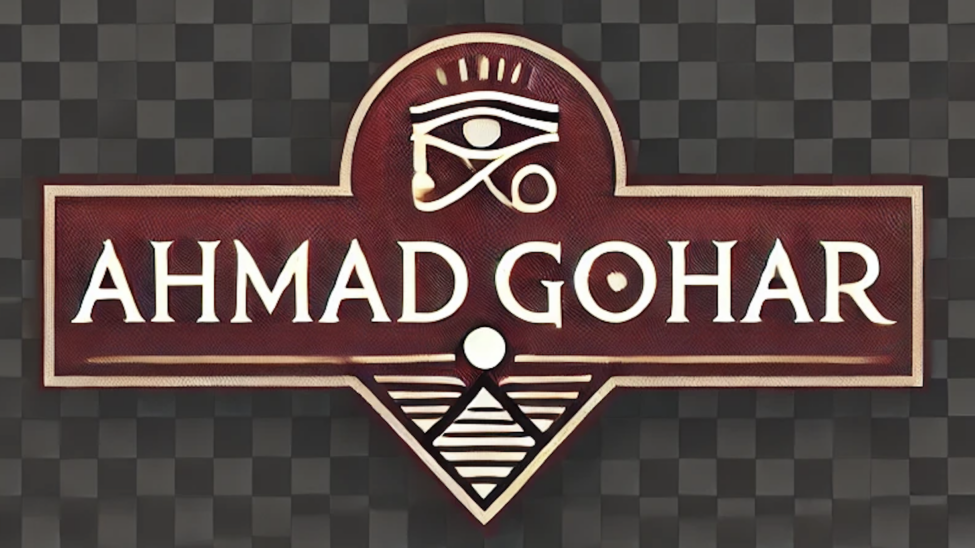
Mastering Bottom-Up Web Services Development: Advantages & Challenges
Understanding Bottom-Up Web Services Development
The bottom-up approach for Web services development begins with existing Java code and generates a Web service interface (WSDL). This method is widely supported by Web services tools and provides an efficient way to expose legacy applications.
Steps in Bottom-Up Development
- Define Data Transfer Objects (DTOs):
- Identify or create JavaBeans to represent the data types for input and output parameters.
- Only attributes with
getter
andsetter
methods are exposed in the Web service interface.
Example:
public class Employee { private int id; private String name; public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } }
- Create a Service Implementation Class:
- Implement the business logic in a
POJO
or a stateless session EJB. - Use DTOs as input and output arguments.
Example:
public class EmployeeService { public Employee getEmployee(int id) { // Business logic to fetch employee } }
- Implement the business logic in a
- Generate Web Service Implementation:
- Use tools like Apache Axis, JAX-RPC, or IDE-integrated wizards to create:
- WSDL file
- Complex type definitions in the
types
element for DTOs - Operations in the
portType
for public methods
- Use tools like Apache Axis, JAX-RPC, or IDE-integrated wizards to create:
- Generate Web Service Client Artifacts:
- Generate client-side DTOs, service endpoint interfaces (SEI), and stubs using the WSDL.
- Use these artifacts to build the client application.
Evaluation of the Bottom-Up Approach
Advantages:
- Quick to Implement:
- Ideal for exposing existing or legacy implementations as Web services.
- Tool-Driven Automation:
- Tools automatically generate WSDL and deployment artifacts, requiring minimal knowledge of WSDL or XML.
- Excellent Tool Support:
- Sophisticated tools streamline both server-side implementation and client-side access.
Disadvantages:
- Schema Generation Limitations:
- Generated schema derives from Java classes, not standards-based schemas, making reuse harder.
- Embedded Schema Challenges:
- Schema types are embedded in the WSDL, complicating reuse in other Web services.
- Limited Parallel Development:
- Client-side development must wait for server-side WSDL generation, delaying parallel workstreams.
- Incremental Change Issues:
- Changes to the server-side class can regenerate the WSDL, potentially breaking compatibility with existing clients.
- Namespace Dependency:
- Embedded schema namespaces are often based on Java package names, leading to incompatibilities if package names change.
Use Cases for Bottom-Up Development
- Exposing Legacy Code: When you need to rapidly expose existing Java code as a Web service.
- Simpler Integration: Ideal for services with straightforward interfaces and minimal schema reuse requirements.
- Tool-Driven Projects: When leveraging tools that automate WSDL and artifact generation.
Conclusion
The bottom-up approach offers a quick and tool-friendly way to expose existing Java code as Web services, but it comes with challenges like schema reuse and managing changes. By understanding the pros and cons, you can decide if this approach fits your project requirements.
Would you like to explore top-down Web services development or discuss integrating bottom-up services into your architecture? 😊