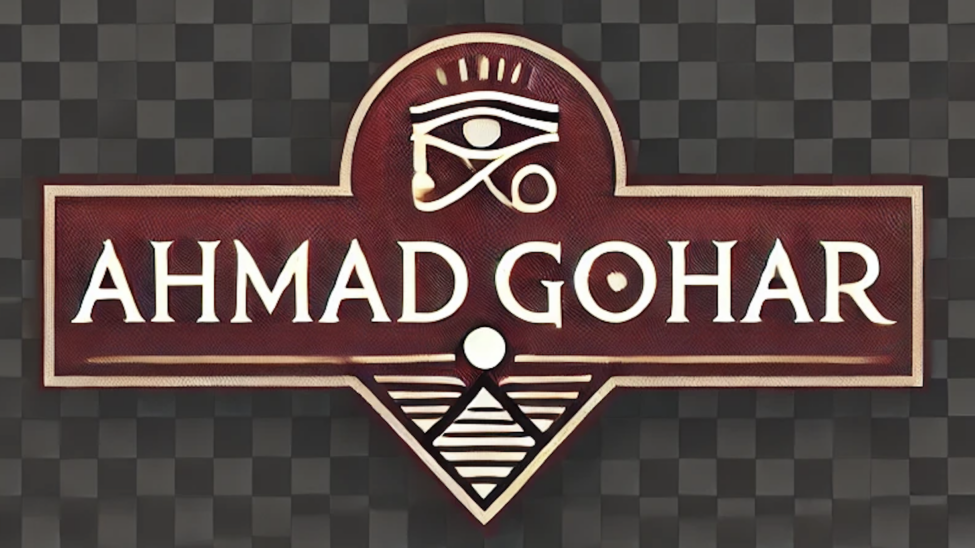
SQL Query Results Cache: Usage, Restrictions, and Best Practices
Understanding SQL Query Result Cache
The SQL Query Result Cache is a feature that improves query performance by storing query results in memory. When enabled, subsequent executions of the same query can retrieve the cached result, avoiding redundant computation or disk reads. Let’s explore its capabilities, rules, and limitations.
Key Facts About SQL Query Result Cache
- Configuration Levels:
- The cache can be enabled or disabled at the system, session, or query level.
- Example:
ALTER SYSTEM SET RESULT_CACHE_MODE = FORCE; -- At system level ALTER SESSION SET RESULT_CACHE_MODE = FORCE; -- At session level SELECT /*+ RESULT_CACHE */ * FROM employees; -- At query level
- Invalidation of Cached Results:
- Cached results become invalid when the underlying data is modified by DML (INSERT, UPDATE, DELETE) operations.
- Conditions for PL/SQL Function Caching:
- The function must not be defined in an anonymous block.
- The function must have at least one
OUT
orIN OUT
parameter.
Query Types That Cannot Be Cached
Certain queries cannot utilize the SQL Query Result Cache due to their dynamic or non-deterministic nature:
- Subquery Results:
- Results of subqueries are not cached.
- Queries Using Non-Deterministic Functions:
- Queries with functions like
SYSDATE
orSYS_TIMESTAMP
are excluded from caching.
- Queries with functions like
- Queries on Dictionary or Temporary Tables:
- Caching is not applied to queries involving dictionary or temporary tables.
- Dynamic SQL:
- Results involving dynamically constructed SQL statements are not cached.
Caching for PL/SQL Functions
When a PL/SQL Function’s Result Is Cached:
- If it is invoked with a parameter value for the first time in the session.
- When RELIES_ON clause specifies tables or views the function depends on, and no DML operation has invalidated the cache.
Example:
CREATE OR REPLACE FUNCTION get_employee_name (p_id NUMBER)
RETURN VARCHAR2
RESULT_CACHE RELIES_ON (employees) IS
BEGIN
RETURN (SELECT first_name || ' ' || last_name
FROM employees
WHERE employee_id = p_id);
END;
/
When Results Are Invalidated:
- A session executes DML on tables or views specified in the RELIES_ON clause.
Benefits of SQL Query Result Cache
- Performance Improvement:
- Reduces database load by reusing cached results.
- Memory Optimization:
- Stores results in shared memory, accessible across sessions.
- Flexibility:
- Can be selectively enabled or disabled for queries or functions.
Limitations of SQL Query Result Cache
Limitation | Details |
---|---|
Non-Deterministic Queries | Queries using SYSDATE , SYS_TIMESTAMP , or similar functions are excluded. |
DML Invalidations | Cache is invalidated upon DML operations on dependent tables. |
Temporary and Dictionary Tables | Queries involving these tables are not cached. |
Dynamic SQL and Subqueries | Results of dynamic SQL or subqueries cannot be cached. |
Best Practices for Using Result Cache
- Cache Deterministic Queries:
- Use result cache for static or rarely updated tables.
- Avoid Non-Deterministic Functions:
- Exclude functions like
SYSDATE
andDBMS_RANDOM
.
- Exclude functions like
- Combine With RELIES_ON Clause:
- Explicitly define dependencies for cached PL/SQL functions.
Conclusion
The SQL Query Result Cache is a powerful tool for optimizing query performance, but its effectiveness depends on understanding its limitations and using it appropriately. By caching deterministic queries and following best practices, you can significantly enhance database efficiency.
Would you like to dive deeper into examples or advanced use cases of SQL Query Result Cache? 😊