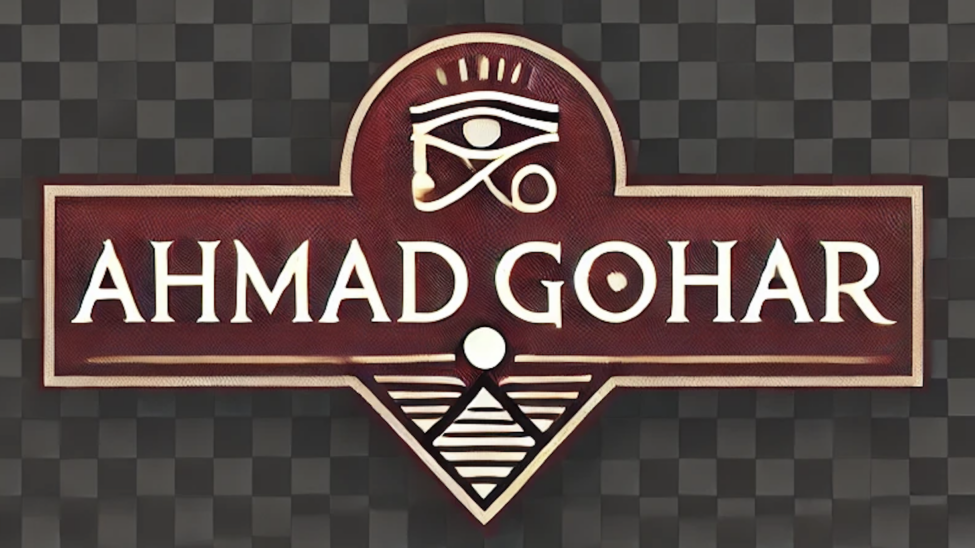
Master Java Labeled Break Statements with Simple Examples
The break statement in Java is a powerful control statement used to terminate loops or switch-case statements. It comes in two forms: unlabeled and labeled. While the unlabeled form is common, the labeled form is particularly useful when dealing with nested loops.
1. Unlabeled Break Statement
An unlabeled break statement ends the nearest enclosing loop (e.g., for
, while
, or do-while
). Here’s an example:
for (int i = 0; i < 10; i++) {
if (i == 5) {
break; // Exits the loop when i equals 5
}
System.out.println("Value: " + i);
}
Output:
Value: 0
Value: 1
Value: 2
Value: 3
Value: 4
In this example, the loop terminates as soon as i
reaches 5.
2. Labeled Break Statement
The labeled break statement is particularly useful in nested loops. It allows you to terminate not just the inner loop but also the outer loop or any specific loop marked with a label.
Syntax:
labelName:
for (/* loop condition */) {
for (/* inner loop condition */) {
if (/* condition */) {
break labelName; // Exits the labeled loop
}
}
}
Example:
outer:
for (int i = 0; i < 3; i++) {
inner:
for (int j = 0; j < 3; j++) {
if (j == 2) {
break inner; // Exits the inner loop
} else if (i == 1) {
break outer; // Exits the outer loop
}
System.out.println("i: " + i + ", j: " + j);
}
}
Output:
i: 0, j: 0
i: 0, j: 1
Key Points to Remember
- Unlabeled Break: Only affects the nearest loop or switch-case block.
- Labeled Break: Targets a specific loop identified by its label.
- Use labeled breaks sparingly, as they can make the code harder to read. Instead, consider refactoring complex loops into smaller, manageable methods.
By understanding and effectively using labeled break statements, you can handle nested loops more efficiently, making your Java programs robust and easier to maintain.
Would you like more examples or guidance on Java control statements? 😊