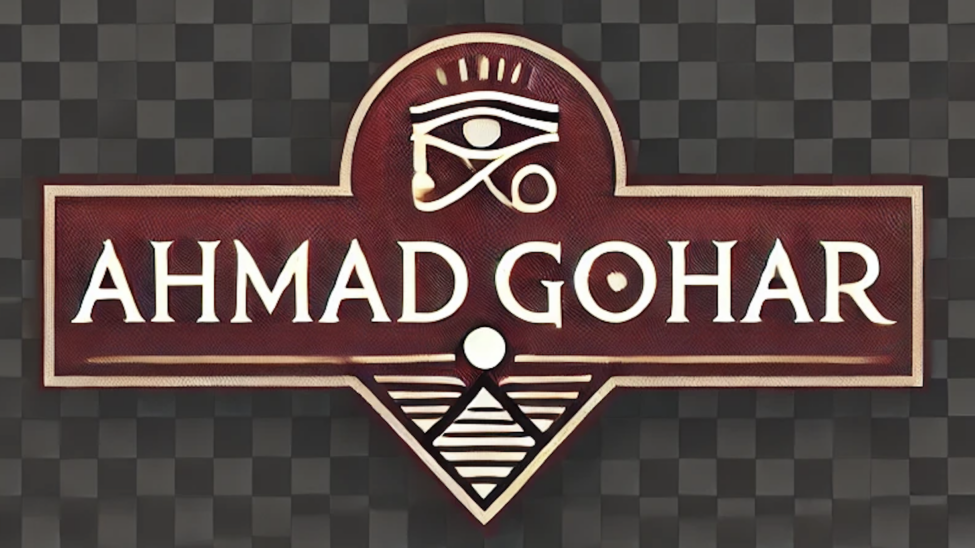
Understanding Forward Declarations in Oracle PL/SQL Packages
Forward Declarations in Oracle PL/SQL: Explanation and Use Cases
Oracle PL/SQL does not allow forward references, meaning that you must declare subprograms before using them. However, certain scenarios, such as maintaining alphabetical order or mutually recursive subprograms, require forward declarations to resolve references.
What Are Forward Declarations in PL/SQL?
A forward declaration is a subprogram specification (heading) terminated by a semicolon that appears before the subprogram body. It allows you to reference a subprogram before its implementation.
Benefits of Forward Declarations:
- Logical Organization: Maintain subprograms in alphabetical or logical order.
- Mutual Recursion: Support subprograms that call each other directly or indirectly.
- Readability: Group related subprograms logically in the package body.
Example of Forward Declaration
Here’s an example demonstrating forward declarations in a package body:
Package Specification
CREATE OR REPLACE PACKAGE PL_TEST AS
-- Public Procedure Declaration
PROCEDURE CALLER;
END PL_TEST;
/
Package Body
CREATE OR REPLACE PACKAGE BODY PL_TEST AS
-- Forward Declaration
PROCEDURE CALC(NUM IN NUMBER);
-- Public Procedure Implementation
PROCEDURE CALLER AS
BEGIN
-- Reference Resolved via Forward Declaration
CALC(100);
END CALLER;
-- Subprogram Implementation
PROCEDURE CALC(NUM IN NUMBER) AS
BEGIN
DBMS_OUTPUT.PUT_LINE('Calculated number: ' || NUM);
END CALC;
END PL_TEST;
/
Key Points:
- Forward Declaration:
PROCEDURE CALC(NUM IN NUMBER);
Declares the subprogram before it’s called inCALLER
. - Subprogram Body:
The implementation ofCALC
appears afterCALLER
.
When to Use Forward Declarations
- Logical or Alphabetical Organization:
If coding standards require procedures to be listed alphabetically, forward declarations allow you to resolve references while maintaining order. - Mutually Recursive Subprograms:
Use forward declarations for subprograms that invoke each other directly or indirectly. - Private Subprograms in Package Bodies:
Forward declarations are needed for private subprograms in the package body if they are referenced before their implementation.
Best Practices
- Consistency in Parameters:
Ensure that the formal parameters in the forward declaration and the subprogram body match exactly in type and order. - Use in Package Body Only:
Public subprogram declarations in the package specification do not require forward declarations. - Organize Subprograms Logically:
Leverage forward declarations to group related subprograms or maintain an alphabetical structure.
Practical Example: Mutually Recursive Subprograms
Scenario:
Two procedures, PROC_A
and PROC_B
, call each other. Forward declarations are required.
CREATE OR REPLACE PACKAGE BODY PL_RECURSION AS
-- Forward Declaration
PROCEDURE PROC_B;
-- Procedure A Implementation
PROCEDURE PROC_A AS
BEGIN
DBMS_OUTPUT.PUT_LINE('Calling PROC_B...');
PROC_B;
END PROC_A;
-- Procedure B Implementation
PROCEDURE PROC_B AS
BEGIN
DBMS_OUTPUT.PUT_LINE('Calling PROC_A...');
PROC_A;
END PROC_B;
END PL_RECURSION;
/
Execution:
BEGIN
PL_RECURSION.PROC_A;
END;
/
Output:
Calling PROC_B...
Calling PROC_A...
Calling PROC_B...
...
Conclusion
Forward declarations in Oracle PL/SQL resolve reference issues while enabling logical or alphabetical organization of subprograms. They are particularly useful for private subprograms in package bodies and mutually recursive subprograms.
Would you like additional examples or clarification on forward declarations in PL/SQL? 😊